javascript
node
Node is a JS runtime environment.
pnpm
My preferred package manager is pnpm. It saves dependencies in one place on disk and hard-links from there on install, resulting in saved space and faster installs.
commitlint | lint git commit |
depcheck | find unused dependencies |
html-validate | validate HTML |
husky | git hooks |
lightningcss | minify CSS |
svgo | optimise SVG |
Vercel | web deployment |
pnpm can also manage Node versions:
pnpm env use --global latest
pnpm env use --global lts
deno
Deno is another and my preferred JS runtime. Having a linter, formatter, and test runner built-in eliminates the need for extraneous dependencies like eslint, prettier, etc.
deps
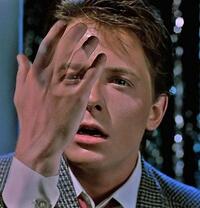
The why reinvent the wheel
and LEGO blocks
counter-arguments to the preference of reducing dependencies break when you wake up one day to some audit alerts, or worse, production issues because of some unpatched bug in a dependency of a dependency of a dependency that has been unmaintained or abandoned for years and all because someone needed lodash just to…check if an array is empty?
It’s understandable for bigger/more complex things, but weaseling your way out of learning how to pick keys from an object or pad a string natively by relying on some library to do it for you is tacky. You’d just be adding a useless beam to what is likely an already needlessly convoluted project.
random
// get a random float between min and max
const rFloat = (min, max) => Math.random() * (max - min) + min
// get a random integer between min and max
const rInt = (min, max) => ~~(Math.random() * (max - min)) + min
// get a random element from an array
const rnArrEl = arr => arr[(Math.random() * arr.length) | 0]
golf
Number(x) === +x
Math.pow(x, y) === (x ** y)
Number.isInteger(x) === (x % 1 === 0)
BigInt(x) === xn
// mind the 32-bit integers ;)
Math.floor(x) === (x >> 0) === ~~x
console
console.table(['alice', 'cheshire', 'hatter'])
console.table({id: 1, item: 'teacup'})
let test = false
console.assert(test, 'message shows up')
test = true
console.assert(test, 'message does not show up')
console.time('ID')
/* do something */
console.timeEnd('ID')
Check if user is online
navigator.onLine
window.addEventListener("offline", (e) => { … }
window.addEventListener("online", (e) => { … }
resources
- A handful of reasons JavaScript won’t be available by
()Andy Bell - Anti-JavaScript JavaScript Club
- Common combinators in JS
- If Not React, Then What? by
()Alex Russell - JavaScript dos and donts by Mu-An Chiou ()
- Manage Node versions with pnpm by
()Francesco Menghi - Numbers in JavaScript by
Stephan Houben - Tao of Node by
()Alex Kondov